Adding Doll Blink Animation
We wanted to end the level with something small but creepy that will hopefully surprise our players. We believe that once the final task is complete and no other prompts appear on screen, the player is bound to eventually check the tag for confirmation that they have completed all the tasks.
Because of this, we can set the level’s ending cutscene of the doll suddenly blinking to play as soon as the player closes the tag once the level is complete.
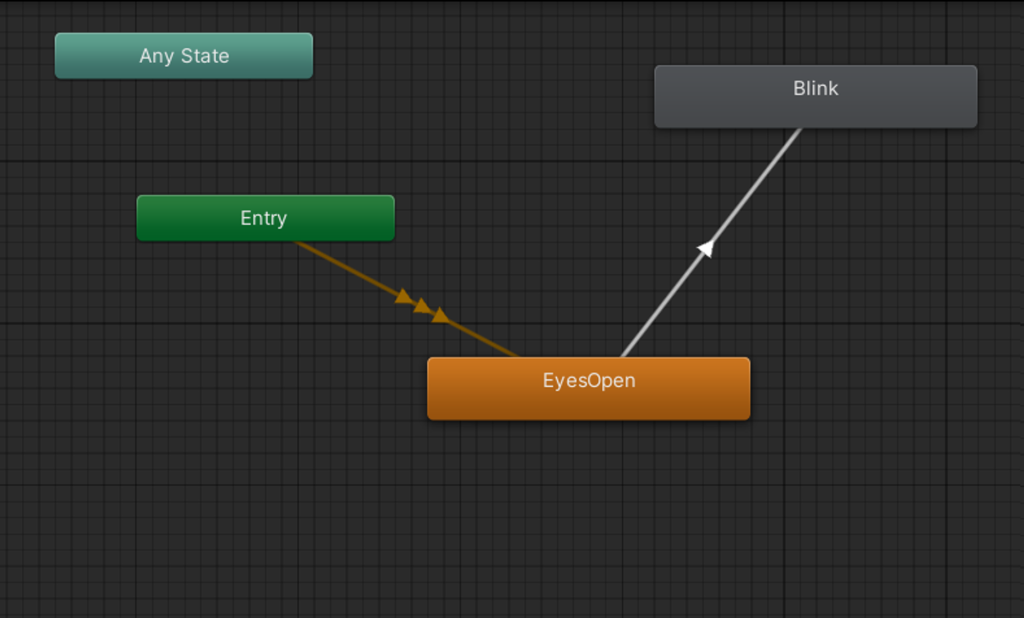
I used Unity’s animation window to set up a blink animation for our doll, using the frames Sam drew for us.
I later realised that I wasn’t sure how to trigger the animation to play at a certain point through a script, and as the sole animation clip in the animator it was set to the default state.
I went back and added a single frame eyes open animation (just the still sprite) as the entry state, and a boolean levelComplete that would cause the animation to transition to the blink state. I could then trigger the animation to play through my script like so:
IEnumerator Wait()
{
anim.SetBool("levelComplete", true);
yield return new WaitForSeconds(3);
animDone = true;
}
I used a coroutine to add a pause pauses/control the flow of the “cutscene”, and make sure the animation has enough time to play before the game loads the next scene.
void Update()
{
if (gm.levelTasks[10].isComplete && gm.levelComplete && !animDone)
{
StartCoroutine(Wait());
}
if (anim.GetBool("levelComplete") == true && animDone)
{
SceneManager.LoadScene("Outro");
}
}
Creating Cutscenes
Sam made some fantastic silent movie videos for us to use as the intro and outro cutscenes for each level.
I implemented cutscenes by creating a very simple scene with nothing but a black backdrop, a video player, and a script for that plays the video on awake and loads the next scene when the video is done. The black backdrop is there so that the blank Unity void doesn’t potentially show up for a second when scenes are loading. The videos render straight to the main camera, making them full-screen.
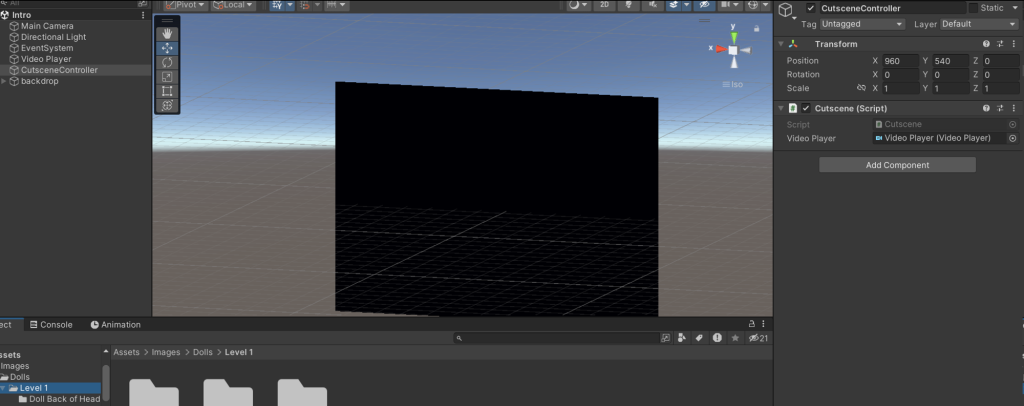
We use Unity’s scene management to get the name of our current scene, and depending on the result, I branch to load the next scene we want.
void Start()
{
Cursor.visible = false;
videoTime = (float)videoPlayer.length;
Invoke("videoEnded", videoTime);
}
void videoEnded()
{
if (SceneManager.GetActiveScene().name == "Intro")
{
SceneManager.LoadScene("Level 1");
}
else if (SceneManager.GetActiveScene().name == "Outro")
{
SceneManager.LoadScene("Main Menu");
}
}

All that’s left after this is to make sure to add any new scenes to our project’s build settings.
For each new cutscene I can just duplicate this scene and replace the video, the update the cutscene script.
Laura Alford